RESTful API는 Representational State Transfer의 약자로, 웹 서비스를 위한 아키텍처 스타일 중 하나입니다. REST는 HTTP(Hypertext Transfer Protocol)를 기반으로 하며, 자원을 표현하고 상태를 전달하기 위한 간단한 방법을 제공합니다.
- REST (Representational State Transfer)
자원을 이름(자원의 표현)으로 구분하여 해당 자원의 상태(정보)를 주고 받는 모든 것을 의미한다.
자원(resource)의 표현(representation) 에 의한 상태 전달
- 자원: 해당 소프트웨어가 관리하는 모든 것
Ex) 문서, 그림, 데이터, 해당 소프트웨어 자체 등
- 자원의 표현: 그 자원을 표현하기 위한 이름
Ex) DB의 학생 정보가 자원일 때, ‘students’를 자원의 표현으로 정한다.
- REST는 자원(리소스)을 다루기 위한 경량의 아키텍처 스타일로, HTTP 프로토콜을 기반으로 합니다.
- REST는 자원을 URI(Uniform Resource Identifier)로 표현하고, HTTP 메서드(GET, POST, PUT, DELETE 등)를 사용하여 자원에 대한 동작(CRUD Operation)을 정의합니다.
- REST의 주요 특징은 자원, HTTP 메서드, URI, 상태 전달, 표현, 계층 구조 등입니다.
*CRUD Operation
Create : 생성(POST)
Read : 조회(GET)
Update : 수정(PUT)
Delete : 삭제(DELETE)
HEAD: header 정보 조회(HEAD)
- REST API 란
- API: 데이터와 기능의 집합을 제공하여 컴퓨터 프로그램간 상호작용을 촉진하며, 서로 정보를 교환가능 하도록 하는 것
- REST API: REST 기반으로 서비스 API를 구현한 것
- REST API 설계 규칙 (이 밖에 더 많은 설계 규칙이 있습니다!!)
- URI는 자원의 정보를 표시해야 한다.
resource는 동사보다는 명사를, 대문자보다는 소문자를 사용한다
resource의 도큐먼트 이름으로는 단수 명사를 사용해야 한다
resource의 컬렉션 이름으로는 복수 명사를 사용해야 한다
resource의 스토어 이름으로는 복수 명사를 사용해야 한다
GET /Member/1 -> GET /members/1
- 자원에 대한 행위는 HTTP Method(GET, PUT, POST, DELETE) 로 표현한다.
- URI에 HTTML Method가 들어가면 안된다
GET /members/delete/1 -> DELETE /members/1 - URI에 행위에 대한 동사 표현이 들어가면 안된다(CRUD 기능은 URI에 사용하지 않는다.)
GET /members/show/1 -> GET /members/1
GET /members/insert/2 -> POST /members/2 - 경로 부분 중 변하는 부분은 유일한 값으로 대체한다(id는 하나의 특정 resource를 나타내는 고유값이다)
Ex) student를 생성하는 route: POST/students
Ex) id=12인 student를 삭제하는 route: DELETE/students/12
- RESTful API (RESTful Application Programming Interface)
RESTful API는 REST 원칙을 준수하여 설계된 API를 의미합니다.
- 구현 예시
날씨 정보 조회기능을 구현한 예시입니다.
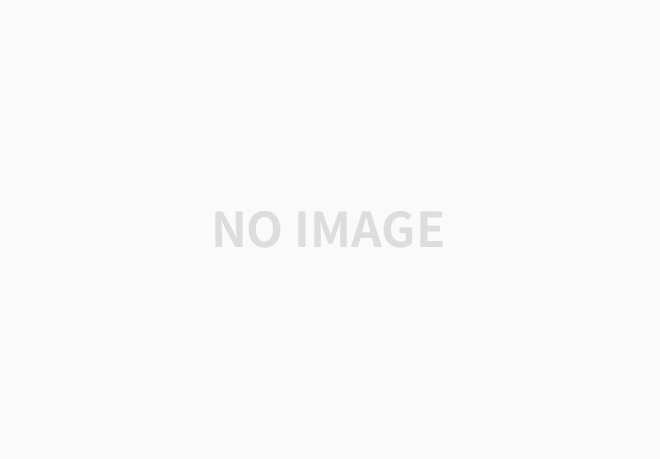
package com.example.demo;
import java.io.Console;
import java.util.List;
import java.util.Optional;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PatchMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import com.example.demo.dao.WeatherTableRepository;
import com.example.demo.entity.WeatherTable;
@RestController
public class WeatherTableController {
private WeatherTableRepository repository;
//스프링부트가 컨트롤러 만들때 레파지토리라는 객체만들어 파라미터로 전달
public WeatherTableController(WeatherTableRepository repository) {
super();
this.repository = repository;
}
@GetMapping("/weather/{id}")
public WeatherTable getWeatherTable (@PathVariable String id) {
return repository.findById(id).orElseThrow(null);
}
@PostMapping("/weather")
public WeatherTable postWeatherTable (@RequestBody WeatherTable weather) {
System.out.println("getpost log :" + weather);
return repository.save(weather);
}
@PutMapping("/weather/{id}")
public WeatherTable putWeatherTable (@PathVariable String id, @RequestBody WeatherTable weather) {
return repository.save(weather);
}
@PatchMapping("/weather/{id}")
public WeatherTable patchWeatherTable (@PathVariable String id, @RequestBody WeatherTable weather) {
return repository.save(weather);
}
@DeleteMapping("/weather/{id}")
public void deleteWeatherTable (@PathVariable String id) {
repository.deleteById(id);
}
@GetMapping("/weather/list")
public List<WeatherTable> getWeatherTableList () {
List<WeatherTable> weatherList = (List<WeatherTable>) repository.findAll();
for(WeatherTable weather : weatherList){
System.out.println("getlist log :" + weather.getId());
}
System.out.println("getlist log :" + weatherList);
return (List<WeatherTable>) repository.findAll();
}
}
Controller
GET, POST, PUT, PATCH, DELETE
요청을 각각 매핑해주는 annotation을 사용하여 구현
URI
자원인 날씨(weather)을 명시함.
필요한 경우 id(key)를 입력 받도록,
전체목록을 가져오는 get요청의 uri에는 list를 추가,
'Frontend > HTTP 통신' 카테고리의 다른 글
쿠키(Cookie), 세션(Session), 캐시(Cache) (0) | 2024.06.05 |
---|---|
[axios] defaults 설정 방법 및 옵션 (0) | 2023.09.14 |
다양한 형태의 axios (0) | 2023.07.18 |
axios post 파라미터 전달 방법 (0) | 2023.05.25 |
List, Array 형태 데이터 파라미터로 Spring 서버와 ajax 통신 (0) | 2022.05.08 |